Building Micronaut Microservices Using MicrostarterCLI
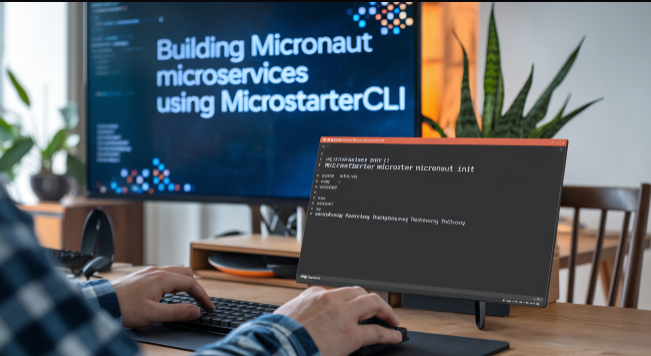
Microservices architecture has gained immense popularity in recent years due to its flexibility, scalability, and ease of development. Among the many frameworks available for building microservices, Micronaut stands out for its performance and efficiency, especially when creating lightweight and reactive microservices. When combined with MicrostarterCLI, developers can accelerate the setup process and ensure consistency across different services.
In this article, we’ll take an in-depth look at building Micronaut microservices using MicrostarterCLI. We will explore how to set up multiple services, configure them for communication, and discuss real-world applications. Additionally, we’ll cover MicrostarterCLI, a command-line interface that simplifies and streamlines microservice development.
1. Introduction to building micronaut microservices using microstartercli
What is Micronaut?
Micronaut is a modern, JVM-based framework designed for building lightweight microservices. Unlike traditional Java frameworks, Micronaut doesn’t rely on runtime reflection, making it faster and more efficient. This makes it a great choice for developers looking to build scalable and high-performance microservices.
Key Features of Micronaut:
- Low memory consumption: Minimal overhead, perfect for microservices.
- Fast startup time: Ideal for applications deployed in cloud environments where startup time matters.
- Cloud-native support: Built-in features for service discovery, configuration management, and integration with cloud services.
- Reactive support: Fully supports reactive programming, enabling developers to build more responsive applications.
What is MicrostarterCLI?
MicrostarterCLI is a command-line tool that helps developers quickly generate code for Micronaut-based applications. It provides standard reusable templates for common microservice setups, such as REST and GraphQL endpoints, service discovery, and configuration management. With MicrostarterCLI, you can automate much of the initial configuration, allowing you to focus on business logic.
Key Features of MicrostarterCLI:
- Rapid code generation: Generates boilerplate code for microservices.
- Configuration templates: Helps you set up standard configurations with minimal effort.
- Modular architecture: Easily add or remove features such as database support or service discovery.
2. Setting Up Your Environment
Before we start building our microservices, you’ll need to set up your development environment. Here’s a quick overview of the tools you’ll need:
Also Read: Exploring the Iconic super mario wonder imagesize:2894×4686
Prerequisites:
- Java Development Kit (JDK): Install JDK 11 or above.
- Micronaut: Install the Micronaut CLI tool for managing your microservices.
- MicrostarterCLI: Download and install MicrostarterCLI from its official repository.
- Gradle or Maven: Both build tools are supported by Micronaut, though Gradle is commonly used.
- Docker: For running services like Eureka and Consul in containers.
- Postman: To test the REST endpoints of our services.
Steps to Install MicrostarterCLI:
- Download MicrostarterCLI from its official GitHub repository.
- Unzip the downloaded file and add it to your system’s PATH.
- Run the command
microstarter --version
to verify the installation.
3. Creating Your First Micronaut Microservice Using MicrostarterCLI
Now that we have the environment set up, let’s create our first Micronaut microservice using MicrostarterCLI. For this example, we will create a Fruit Service—a simple CRUD service to manage a collection of fruit objects.
Step 1: Generate a Micronaut Project
Run the following command to create a new Micronaut microservice project:
bashCopy codemicrostarter create-service fruit-service
This command creates a basic Micronaut microservice with standard configurations, including REST API endpoints.
Step 2: Define the Fruit Entity
Create a simple Fruit
class that represents the fruit object. This class will have properties like id
, name
, and color
.
javaCopy codepublic class Fruit {
private Long id;
private String name;
private String color;
// Getters and Setters
}
Step 3: Set Up CRUD Operations
Using Micronaut’s support for controllers, define CRUD operations for the Fruit
entity in the FruitController
class.
javaCopy code@Controller("/fruits")
public class FruitController {
private final List<Fruit> fruits = new ArrayList<>();
@Get
public List<Fruit> listFruits() {
return fruits;
}
@Post
public HttpResponse<Fruit> addFruit(@Body Fruit fruit) {
fruits.add(fruit);
return HttpResponse.created(fruit);
}
@Delete("/{id}")
public HttpResponse<?> deleteFruit(@PathVariable Long id) {
fruits.removeIf(fruit -> fruit.getId().equals(id));
return HttpResponse.noContent();
}
}
Step 4: Run the Service
Navigate to your project folder and use the following command to run the service:
bashCopy code./gradlew run
Your Fruit Service is now running, and you can access the REST endpoints via tools like Postman or Curl.
4. Understanding the Fruit and Vegetable CRUD Services
With MicrostarterCLI, you can easily add more services following the same pattern as the Fruit Service. Let’s quickly walk through adding a Vegetable Service.
Step 1: Create the Vegetable Service
Run the following command to create a new Vegetable Service:
bashCopy codemicrostarter create-service vegetable-service
Step 2: Define the Vegetable Entity
Create a simple Vegetable
class that mirrors the structure of the Fruit
class.
javaCopy codepublic class Vegetable {
private Long id;
private String name;
private String type;
}
Step 3: Set Up CRUD Operations
The CRUD operations for the Vegetable Service follow the same structure as the Fruit Service, with the necessary modifications for the Vegetable
entity.
Now, with both services up and running, we can move on to service discovery and configuration management.
5. Service Discovery with Eureka
Service discovery is crucial in microservices architecture because it allows services to discover and communicate with each other dynamically. Eureka is a popular service discovery tool that integrates seamlessly with Micronaut.
Step 1: Set Up Eureka
To use Eureka, we first need to start a Eureka Server. You can either run it in a Docker container or set it up manually. Here’s how to run it with Docker:
bashCopy codedocker run -d --name eureka -p 8761:8761 eureka-server
Step 2: Configure Micronaut Services for Eureka
In each service (Fruit and Vegetable), you’ll need to add the Eureka client dependency to the build.gradle
file:
gradleCopy codeimplementation("io.micronaut:micronaut-discovery-client")
Then, configure Eureka in the application.yml
file of each service:
yamlCopy codeeureka:
client:
serviceUrl:
defaultZone: http://localhost:8761/eureka/
After restarting the services, they will automatically register with Eureka.
6. Configuration Management Using Consul
Consul is a popular tool for configuration management in microservices architectures. It allows us to manage configuration files centrally and dynamically update services as needed.
Step 1: Set Up Consul
You can run Consul using Docker:
bashCopy codedocker run -d --name consul -p 8500:8500 consul
Step 2: Configure Micronaut Services for Consul
To integrate Consul with your services, add the following dependency in build.gradle
:
gradleCopy codeimplementation("io.micronaut:micronaut-discovery-client-consul")
Then, configure the application.yml
file to point to the Consul server:
yamlCopy codemicronaut:
application:
name: fruit-service
consul:
client:
host: localhost
port: 8500
Once configured, your Micronaut microservices can retrieve and update their configuration from Consul.
7. Spring Cloud Gateway Integration
In a microservices architecture, we often need a gateway to route requests to different services. Spring Cloud Gateway is a lightweight gateway solution that works well with Micronaut.
Step 1: Set Up Spring Cloud Gateway
Add the Spring Cloud Gateway dependencies to the gateway service’s build.gradle
file:
gradleCopy codeimplementation("org.springframework.cloud:spring-cloud-starter-gateway")
Step 2: Configure Routes
In the application.yml
of the gateway service, define routes for your Fruit and Vegetable services:
yamlCopy codespring:
cloud:
gateway:
routes:
- id: fruit-service
uri: http://localhost:8080/fruits
predicates:
- Path=/fruits/**
- id: vegetable-service
uri: http://localhost:8081/vegetables
predicates:
- Path=/vegetables/**
With the gateway set up, requests can be routed to the correct service based on the URL path.
8. Testing and Deploying Micronaut Microservices
Testing is an essential part of the development process, especially in microservices. Here’s how to test and deploy your Micronaut microservices:
Unit Testing
Micronaut provides built-in support for testing. Create test cases for your services using the JUnit framework.
javaCopy codeimport io.micronaut.test.annotation.MicronautTest;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
@MicronautTest
class FruitControllerTest {
@Test
void testFruitCreation() {
// Test logic here
}
}
Deployment Options
- Docker: Package your services as Docker containers and deploy them on a cloud platform like AWS or Google Cloud.
- Kubernetes: For managing large-scale deployments, use Kubernetes to orchestrate and manage your services.
FAQs About building micronaut microservices using microstartercli
1. What is Micronaut?
Micronaut is a JVM-based framework designed for building lightweight, scalable microservices that support reactive programming.
2. What is MicrostarterCLI?
MicrostarterCLI is a command-line tool that helps developers quickly generate reusable code templates and configurations for Micronaut microservices.
3. Why use Eureka for service discovery?
Eureka allows microservices to discover and communicate with each other dynamically, which is essential for scalability.
4. How does Consul help with configuration management?
Consul provides centralized configuration management, allowing microservices to update their configurations dynamically without restarting.
5. What is the role of Spring Cloud Gateway?
Spring Cloud Gateway acts as a reverse proxy, routing incoming requests to the appropriate microservice based on predefined routes.
6. How can I test my Micronaut microservices?
Micronaut provides built-in support for unit and integration testing using JUnit, allowing you to test your services effectively.
Conclusion Of building micronaut microservices using microstartercli
By following this comprehensive guide, you can easily set up Micronaut microservices using MicrostarterCLI, configure them for service discovery and configuration management, and route requests using Spring Cloud Gateway. With these steps, you can ensure that your microservices architecture is efficient, scalable, and ready for production.